Why is it necessary to add ads.txt?
Authorized Digital Sellers (aka ads.txt) is an IAB Tech Lab initiative that helps ensure that your digital ad inventory is only sold through sellers (such as AdSense) who you've identified as authorized.
Creating your own ads.txt file gives you more control over who's allowed to sell ads on your site and helps prevent counterfeit inventory from being presented to advertisers.
The requirement is as simple as, download the ads.txt file and then drop it on your root. This file must be accessible when you go to www.venopi.com/ads.txt (Venopi is my startup, so we're using this as sample). However, it doesn't work like that on your Django project. Simply dropping this ads.txt file on your root doesn't automagically mean that it's accessible on www.venopi.com/ads.txt - So how?
But of course, download your ads.txt file
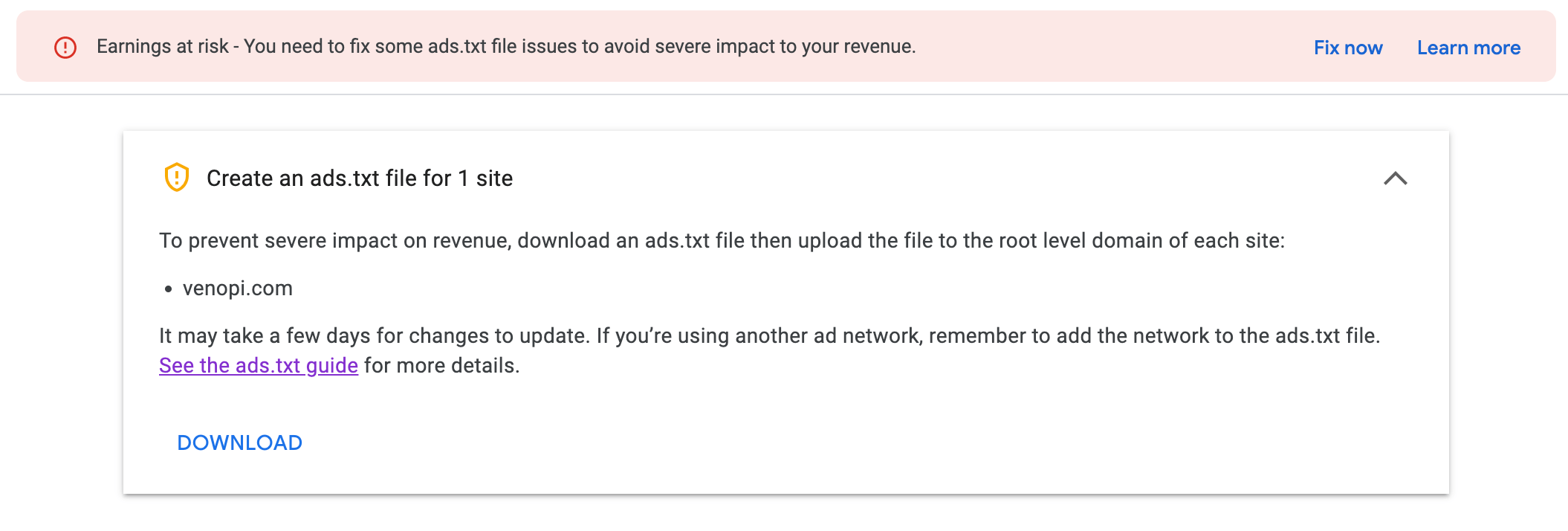
Solution: Put ads.txt on your static folder and redirect
Go to your static folder and drop ads.txt file there. If you cannot find your static folder, check your `settings.py` and search for the static path:
STATIC_URL = '/static/'
STATICFILES_DIRS = (
os.path.join(BASE_DIR, "static"),
)
When you do this, you can already access the ads.txt file when you go to www.venopi.com/static/ads.txt. However as mentioned on the Adsense documentation, the right path where Adsense will check is www.venopi.com/ads.txt
from django.views.generic import RedirectView
from django.contrib.staticfiles.storage import staticfiles_storage
from django.conf.urls import include, url
from django.conf import settings
from django.conf.urls.static import static
urlpatterns = [
// other urls...
url(r'^ads.txt', RedirectView.as_view(url=staticfiles_storage.url("ads.txt"))),
] + static(settings.STATIC_URL, document_root=settings.STATIC_ROOT) + \
static(settings.MEDIA_URL, document_root=settings.MEDIA_ROOT)
You can now try to visit www.venopi.com/ads.txt and you will be redirected to www.venopi.com/static/ads.txt 🎉
Don't like the redirect solution? OK, we have another solution!
Solution: Create a new View
Create a new class inside one of your views. It doesn't matter where, as long as you reference it correctly on your `urls.py`
from django.views.generic import View
class Adsense(View):
def get(self, request, *args, **kwargs):
return HttpResponse('google.com, pub-REPLACE_WITH_YOUR_PUB_ID, DIRECT, f08c47fec0942fa0')
On `urls.py`, you need to import the `Adsense class` like so:
from common.views import Adsense
urlpatterns = [
// other urls...
url(r'ads.txt', Adsense.as_view()),
] + static(settings.STATIC_URL, document_root=settings.STATIC_ROOT) + \
static(settings.MEDIA_URL, document_root=settings.MEDIA_ROOT)
Any questions or suggestions for better implementations? Write to me 😀